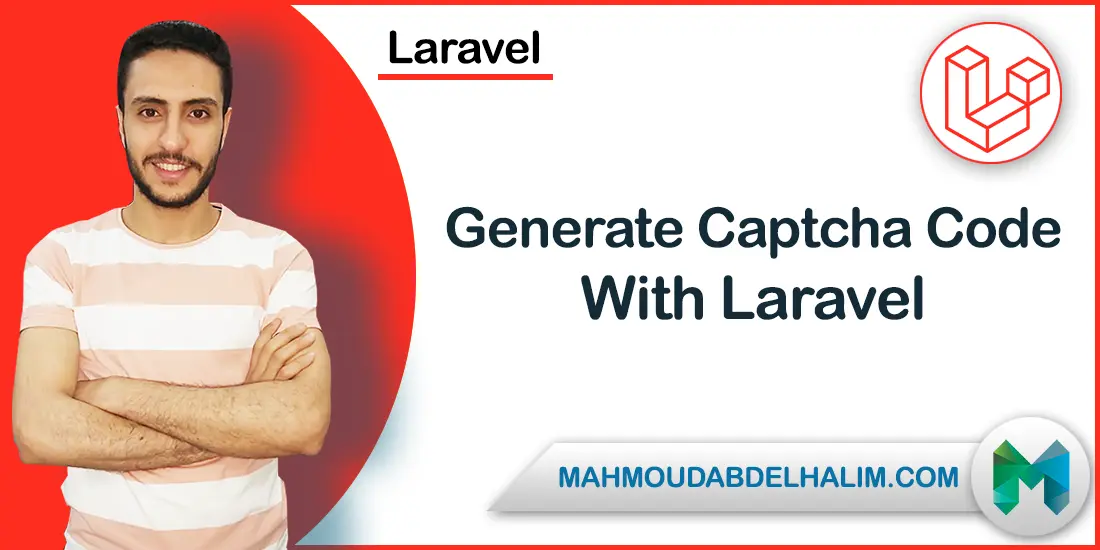
I think we should use the captcha code on our registration form because the captcha code prevents spam, bots, etc. In most of the applications, we need to use captcha verification because it is very important for security reasons.
In most of the applications, we need to use captcha verification because it is very important for security reasons. There are several libraries to generate captcha images in Laravel. In this example, I use the BotDetect package library to generate captcha images and use their validation. BotDetect is a very popular library and a very customized library. they provide several UI designs for captcha code also how many characters do you want? you can set all the things using this package.
Step 1: Installation
In the first step, we will install a captcha package to generate the captcha code image.
composer require captcha-com/laravel-captcha:"4.*"
Now we need to add the provider and alias in the config/app.php file so open that file and add the bellow code in provides array:
LaravelCaptcha\Providers\LaravelCaptchaServiceProvider::class,
to use your own settings to publish the config file.
php artisan vendor:publish
Step 2: Add Routes
In the second step, we will add new two routes for creating a small example so that way we can understand it very well. so first add the bellow route in your routes/web.php file.
Route::get('captcha_register', 'Auth\AuthController@registerWithCaptcha']);
Route::post('captcha_register', 'Auth\AuthController@registerWithCaptchaPost'])->name('captcha_register');
Step 3: Add Controller Method
In this step, We will create this file (AuthController.php) and we will add registerWithCaptcha()
and registerWithCaptchaPost()
methods in the app/Http/Controllers/Auth/AuthController.php file for our example.
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Hash;
use Illuminate\Http\Request;
use App\User;
use Auth;
class AuthController extends Controller
{
/**
* Where to redirect users after login.
*
* @var string
*/
protected $redirectTo = '/home';
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('guest')->except('logout');
}
/**
* Create a new controller instance.
*
* @return void
*/
public function registerWithCaptcha()
{
return view('auth.captchaRegister');
}
/**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\User
*/
public function registerWithCaptchaPost(Request $request)
{
$this->validate($request, [
'name' => 'required',
'email' => 'required|email',
'password' => 'required|same:password_confirmation',
'password_confirmation' => 'required',
'CaptchaCode' => 'required|valid_captcha'
]);
print('write your other code here.');
}
}
Step 4: Add Blade Files
In this step, we just create a new blade file captchaRegister.blade.php, and put the bellow code on that file in this path.
@section('content')
<link href="{{ captcha_layout_stylesheet_url() }}" type="text/css" rel="stylesheet">
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">{{ __('Register') }}</div>
<div class="card-body">
<form method="POST" action="{{ route('captcha_register') }}">
@csrf
<div class="form-group row">
<label for="name" class="col-md-4 col-form-label text-md-right">{{ __('Name') }}</label>
<div class="col-md-6">
<input id="name" type="text" class="form-control @error('name') is-invalid @enderror" name="name" value="{{ old('name') }}" required autocomplete="name" autofocus>
@error('name')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<label for="email" class="col-md-4 col-form-label text-md-right">{{ __('E-Mail Address') }}</label>
<div class="col-md-6">
<input id="email" type="email" class="form-control @error('email') is-invalid @enderror" name="email" value="{{ old('email') }}" required autocomplete="email">
@error('email')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label>
<div class="col-md-6">
<input id="password" type="password" class="form-control @error('password') is-invalid @enderror" name="password" required autocomplete="new-password">
@error('password')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<label for="password-confirm" class="col-md-4 col-form-label text-md-right">{{ __('Confirm Password') }}</label>
<div class="col-md-6">
<input id="password-confirm" type="password" class="form-control" name="password_confirmation" required autocomplete="new-password">
</div>
</div>
<div class="form-group row">
<label for="CaptchaCode" class="col-md-4 col-form-label text-md-right">Captcha</label>
<div class="col-md-6">
{!! captcha_image_html('ExampleCaptcha') !!}
<input id="CaptchaCode" type="text" class="form-control @error('CaptchaCode') is-invalid @enderror" name="CaptchaCode" style="margin-top:5px;" required>
@if ($errors->has('CaptchaCode'))
<span class="invalid-feedback">
<strong>{{ $errors->first('CaptchaCode') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-6 offset-md-4">
<button type="submit" class="btn btn-primary">
{{ __('Register') }}
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
@endsection
This is after adding the blade:
Step 5: Create User Finally
This is the last step, we will add the creation code on the registerWithCaptchaPost()
method.
/**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\User
*/
public function registerWithCaptchaPost(Request $request)
{
$this->validate($request, [
'name' => 'required',
'email' => 'required|email',
'password' => 'required|same:password_confirmation',
'password_confirmation' => 'required',
'CaptchaCode' => 'required|valid_captcha'
]);
$user = User::create([
'name' => $request->input('name'),
'email' => $request->input('email'),
'password' => Hash::make($request->input('password')),
]);
Auth::guard()->login($user);
return redirect($this->redirectTo)->with('status','User Created Success..');
}
After running the code, these is the results:
Thanks and I hope it can help you.
Mahmoud Abd Elhalim
I am a professional web developer with years of experience in the web applications and software industry, Follow me to got more..
Comments