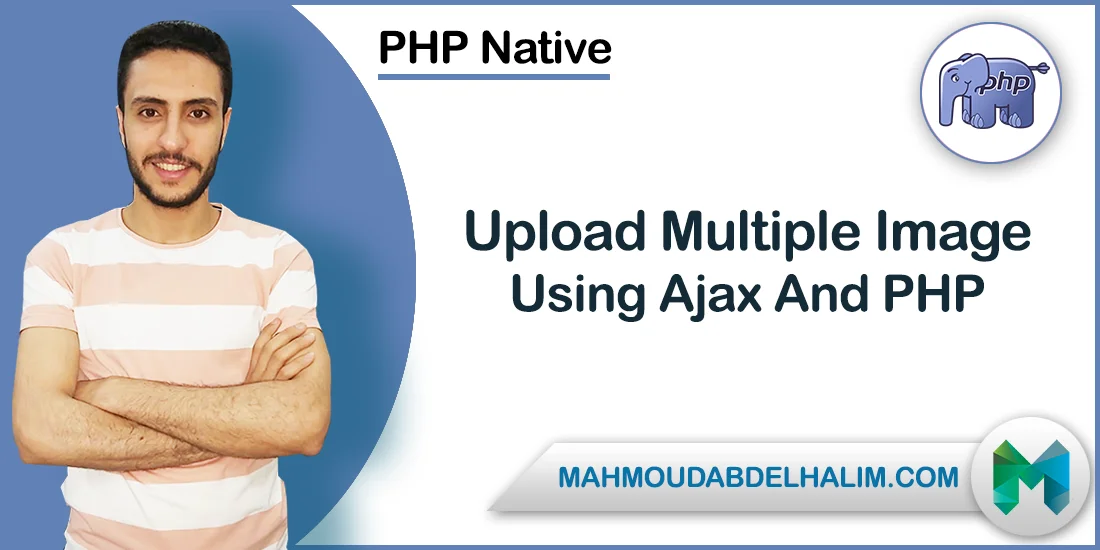
In this tutorial, we have a simple script to Upload Multiple Image Using Ajax With PHP, It is very simple to upload images to the server via ajax() without refreshing the whole page, once an image was uploaded it will be viewed on the same page. PHP code was used at the back-end which will select and upload an image and the uploading process will be done by ajax method.
We will create 3 files, a simple HTML form to upload images files, PHP file to upload files to the server-side, and JS file to upload files easily without reloading page and to view files after uploading.
Step1: Create HTML file.
We will create a simple HTML form to upload the files and send the POST request to the PHP file to handling the uploading process.
- The file name is: index.php
- Add brackets [] to the input name and multiple="" property to allowed upload multi-files.
<!doctype html>
<html>
<head lang="en">
<meta charset="utf-8">
<title>Mahmoud Abd Elhalim</title>
<link rel="stylesheet" href="style.css" type="text/css" />
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/script.js"></script>
</head>
<body>
<div class="container">
<h1><a href="http://mahmoudabdelhalim.com/singleblog/3" target="_blank">Upload Multiple Image Using Ajax</a></h1>
<hr>
<div id="preview"><img src="no-image.jpg" /></div>
<form id="form" action="upload.php" method="POST" enctype="multipart/form-data">
<input id="uploadImage" type="file" accept="image/*" name="images[]" multiple="" />
<input id="button" type="submit" value="Upload File">
</form>
<div id="error"></div>
<hr>
<p><a href="http://www.mahmoudabdelhalim.com" target="_blank">www.mahmoudabdelhalim.com</a></p>
</div>
</body>
</html>
Step2: Create PHP file.
We will create a very simple PHP code to upload images to the directory "uploads/", and we have a validation code for the image and it will upload only valid extension images.
- The file name is: upload.php
- In the code, we will check if it was a POST request or not.
- We will make validation on the file and rename it.
// valid extensions
$allowedexts = array('image/jpg', 'image/jpeg','image/png','image/gif');
// upload directory
$dir = 'uploads/';
// Max size file.
$maxsize = 5000000000;
// Random Numbers.
$random = rand(0000, 9999);
// Output..
$output = '';
$error = FALSE;
// Run the code if have request.
if(isset($_FILES['images']))
{
// Make all validation before ploading...
for ($i=0; $i < count($_FILES['images']['name']) ; $i++)
{
$type = $_FILES['images']['type'][$i];
$size = $_FILES['images']['size'][$i];
if ($size > $maxsize)
{
$error = TRUE;
echo "error in size, Max size ".$maxsize;
}
else if (!in_array($type , $allowedexts))
{
$error = TRUE;
echo "invalid extension, the allowed extensions 'jpg, jpeg, png, gif)";
}
}
// Stop runing if found error..
if ($error == TRUE)
{
die;
}
for ($i=0; $i < count($_FILES['images']['name']) ; $i++)
{
$name = $_FILES['images']['name'][$i];
$temp = $_FILES['images']['tmp_name'][$i];
// Other info if you need.
// $type = $_FILES['images']['type'][$i];
// $size = $_FILES['images']['size'][$i];
$nameEdit = strtolower($name);
$fileLetterName = $random."_".date("dmY")."_".$nameEdit;
$destinationLetter = $dir.$fileLetterName;
$done = move_uploaded_file($temp, $destinationLetter);
$output .= "<p>Upload Done </p><img src='$dir/$fileLetterName'/>";
}
echo $output;
}
Step3: Create JS file.
The file will contain the JS (jQuery) code to upload images without reloading the page and view images. The file AJAX method will be sent to the PHP file to upload files.
The file name is: script.js
We used the AJAX method to send POST request and handling the request.
$(document).ready(function (e) {
$("#form").on("submit", function (e) {
e.preventDefault();
$.ajax({
url: "upload.php",
type: "POST",
data: new FormData(this),
contentType: false,
cache: false,
processData: false,
beforeSend: function () {
//$("#preview").fadeOut();
$("#error").fadeOut();
},
success: function (data) {
console.log(data);
if (data == "invalid") {
// invalid file format.
$("#error").html("Invalid File !").fadeIn();
} else {
// view uploaded file.
$("#preview").html(data).fadeIn();
$("#form")[0].reset();
}
},
error: function (e) {
$("#error").html(e).fadeIn();
},
});
});
});
Thanks can you download the source code.
Download Soure Code: Click Here
Mahmoud Abd Elhalim
I am a professional web developer with years of experience in the web applications and software industry, Follow me to got more..
Comments